API Access
Mesa is designed to be a drop-in replacement for the existing public RPC and REST endpoints. This means that you can use Mesa without having to change any of your existing code, except for the use of the API key to authenticate your access.
In this document we will explore how to use Mesa API, as well as authentication and security considerations.
API Endpoints
All Mesa endpoints are composed of two parts (as usual with any other access-based API services):
ACCESS_KEY
is a unique identifier that is used to identify your account is used to authenticate your access, and measure your quota usage.
It is recommended that you keep this key private, unless you are using it for public access, i.e. from a Webapp.
API_ENDPOINT
are a list of endpoints that a chain supports. Cosmos-based blockchains usually implement 3 endpoints; rpc
, rest
and grpc
.
All blockchains Mesa support have support for all these three endpoints, with a near 100% compatibility.
The list of endpoints for each chain can be found in the Dashboard > Mesa > Registry.
Default endpoints
Default endpoints are the endpoints where your ACCESS_KEY
is part of the URL as a subdomain.
This allows you to quickly access the endpoints without having to alter request headers (i.e. using the Authorization
header in the HTTP request).
In most cases, you can use the default endpoints for accessing. This is the easiest way to access Mesa endpoints.
Endpoint Type | Description | Original Port | Serving Port | URL | TLS |
---|---|---|---|---|---|
RPC | CometBFT RPC | 26657 | 443 | https://<access-key> .<chainID> .mesa-rpc.newmetric.xyz | Yes |
REST | Cosmos SDK API | 1317 | 443 | https://<access-key> .<chainID> .mesa-rest.newmetric.xyz | Yes |
gRPC | CosmosSDK gRPC | 9090 | 443 | <access-key> .<chainID> .mesa-grpc.newmetric.xyz:443 | Yes |
RPC/REST endpoints are served with TLS (HTTPS), but gRPC points are served with plaintext
.
This is a convenience feature. Most Cosmos gRPC libraries assume that the default transport is plaintext
. On top of this, gRPC with TLS is known to add a significant overhead to the performance.
Subdomain-based Authorization is a convenience feature for Webapps. While it is easy to setup,
it is leaking your ACCESS_KEY
to the public, which may be exploited by malicious actors.
It is recommended to setup proper Access Control if you wish to use this feature.
Getting your API Key
You can find your API key in the Dashboard > Mesa > Registry.
Select the chain you want to access, and all available endpoints along with your ACCESS_KEY
will be displayed. Click on the Copy
button to copy the ACCESS_KEY
to your clipboard.
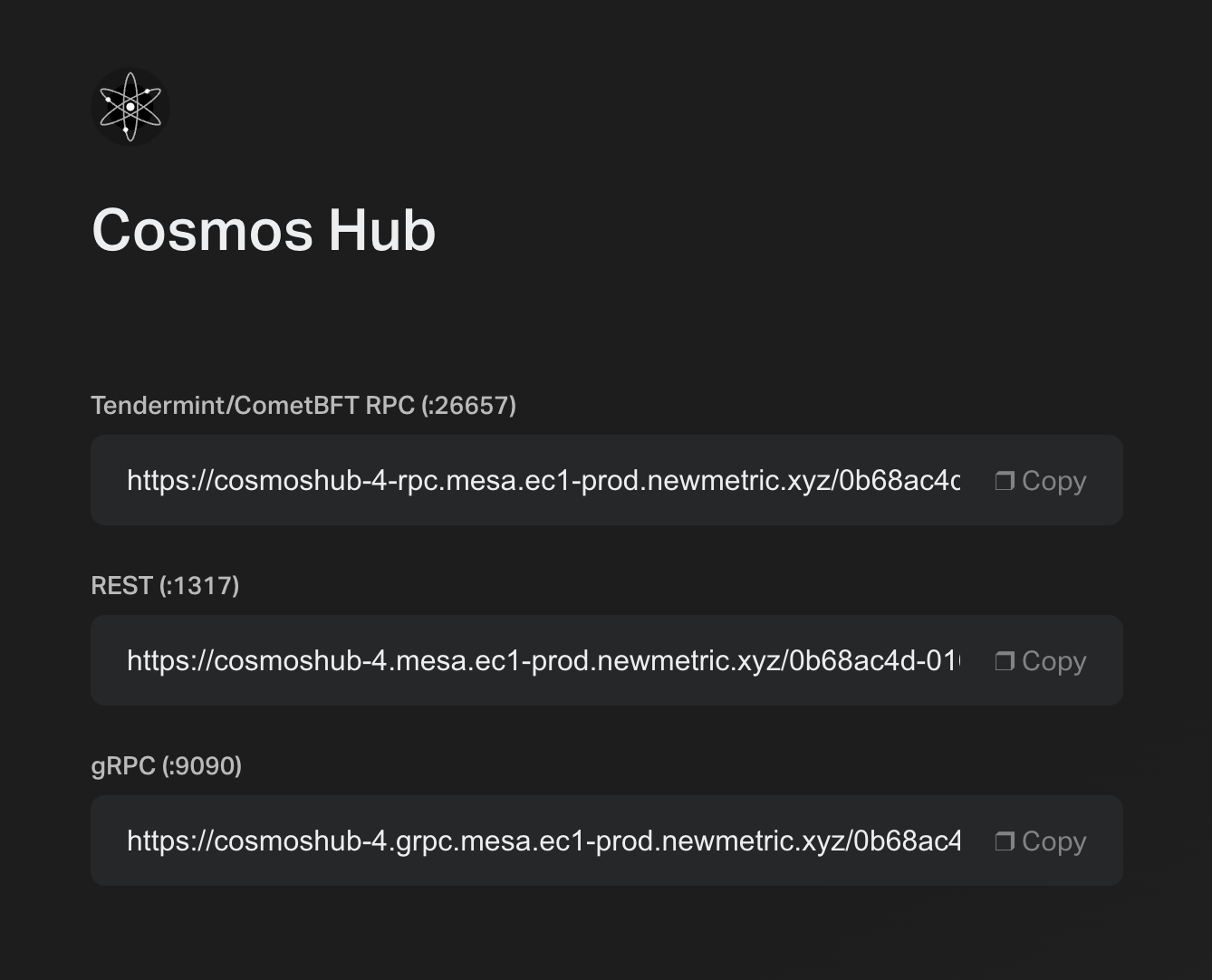
Examples
Example: Latest block
One of the most common use cases is to get the latest block. Here we will demonstrate how to get the latest block using endpoints from all 3 endpoint types.
- For
rpc
andrest
endpoints, we are usingcurl
to demonstrate the usage of the endpoints. - For
gRPC
endpoints, we are usinggrpcurl
to demonstrate the usage of gRPC endpoints.
$ export ACCESS_KEY=<accessKey>
# using rpc
$ curl https://$ACCESS_KEY.cosmoshub-4.mesa-rpc.newmetric.xyz/block \
> {"jsonrpc":"2.0","id":-1,"result":{"block_id":...
# using rest
$ curl https://$ACCESS_KEY.cosmoshub-4.mesa-rest.newmetric.xyz/cosmos/base/tendermint/v1beta1/blocks/latest \
> {"jsonrpc":"2.0","id":-1,"result":{"block_id":...
# using gRPC
$ grpcurl \
$ACCESS_KEY.cosmoshub-4.mesa-grpc.newmetric.xyz:443 \
cosmos.base.tendermint.v1beta1.Service/GetLatestBlock
Example: Getting account balance
Another common use case is to get the balance of an account. Here is an example of how to get the balance of an account using both rest
and gRPC
endpoints.
$ export ACCESS_KEY=<accessKey>
$ export ACCOUNT=cosmos1.... # random account
# using rest
$ curl https://$ACCESS_KEY.cosmoshub-4.mesa-rest.newmetric.xyz/cosmos/bank/v1beta1/balances/$ACCOUNT \
> {"balances": [...]}
# using gRPC
$ grpcurl \
-d "{\"address\":\"$ACCOUNT\"}" -v \
$ACCESS_KEY.cosmoshub-4.mesa-grpc.newmetric.xyz:443 \
cosmos.bank.v1beta1.Query/AllBalances
> {"balances": [...]}
Example: Web Access using Javascript
Because all Mesa endpoints are fully HTTP(S) compatible, you can use them directly from your webapp, using any HTTP client library. Here is an example of how to get the latest block using Javascript.
const ACCESS_KEY = "<accessKey>";
const API_RESOURCE = "block";
// using subdomain-based authorization; use your API key as subdomain,
// followed by the resource you want to access
const API_URL = `https://${ACCESS_KEY}.cosmoshub-4.mesa-rpc.newmetric.xyz/block`;
const response = await fetch(API_URL).then((response) => response.json());
console.log(response);
Advanced Security Considerations
The default endpoints expose your access key in the URL. This means that anyone who has access to the URL (i.e. Webapp users) can see your API key, and may exploit your quota usage.
Generally, it is a good practice that you limit the permissions of the API key to only certain domains, or referers
, that you wish to allow accesses from.
Limit API access
You can limit the access of your API key from the Dashboard > Mesa > Access Control. Refer to the following section for more details.
Header based authorization
You may choose to use header-based authorization
, instead of subdomain-based authorization
.
Technically, this still "leaks" the ACCESS_KEY
to the users through the request header.
However, this type of access is a little more secure because ACCESS_KEY
is only visible to the client and our authorization servers.
To use header authorization:
- Use the following endpoints; they are essentially the same endpoints without the
ACCESS_KEY
as subdomain. - Pass
ACCESS_KEY
as part of theAuthorization
header, withBearer
token type.
We recommend combining this usage with Access Control for better security.
Endpoint Type | Description | Original Port | Serving Port | URL | TLS |
---|---|---|---|---|---|
RPC | CometBFT RPC | 26657 | 443 | https://<chainID> .mesa-rpc.newmetric.xyz | Yes |
REST | Cosmos SDK API | 1317 | 443 | https://<chainID> .mesa-rest.newmetric.xyz | Yes |
gRPC | Cosmos SDK gRPC | 9090 | 443 | <chainID> .mesa-grpc.newmetric.xyz:443 | Yes |
$ export $ACCESS_KEY=<accessKey>
# Authorizing using header
$ curl https://cosmoshub-4.mesa-rpc.newmetric.xyz/block \
-H 'Authorization: Bearer $ACCESS_KEY'
# Authorizing gRPC using access key as subdomain
$ grpcurl \
-H "Authorization: Bearer $ACCESS_KEY"
cosmoshub-4.mesa-grpc.newmetric.xyz:443 \
cosmos.base.tendermint.v1beta1.Service/GetLatestBlock